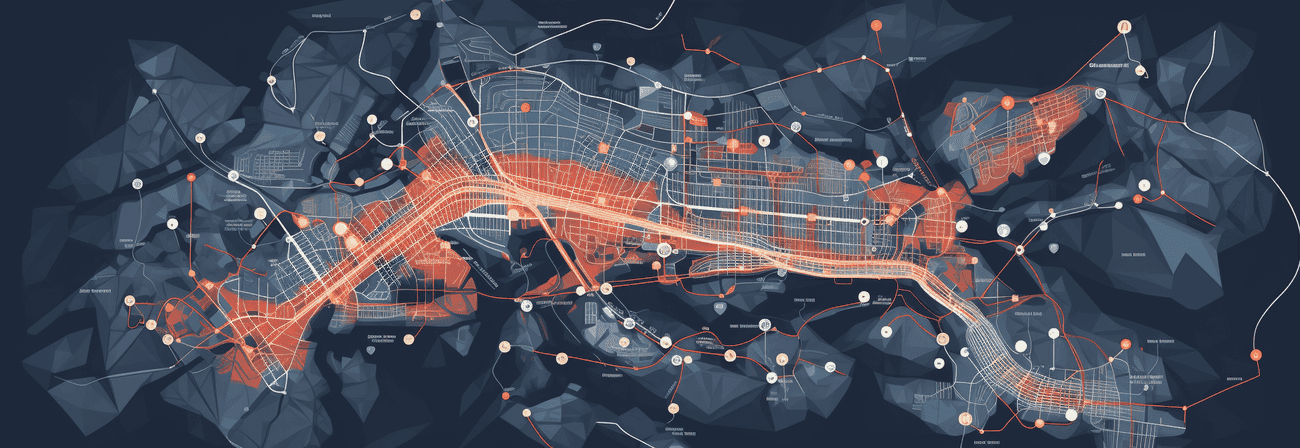
Mastering React and OpenLayers Integration: A Comprehensive Guide
Maps have long been a fundamental element in web development, transforming static websites into dynamic, location-aware applications. Whether you're navigating through the bustling streets of a city, planning a route for your next adventure, or visualizing data in a geographic context, maps play a crucial role in enhancing user experiences.
Overview of OpenLayers and its Capabilities
OpenLayers, a robust open-source JavaScript library, stands at the forefront of enabling you to seamlessly integrate interactive maps into web applications. Its versatile and feature-rich nature makes it a go-to choice for projects requiring dynamic geospatial visualizations.
At its core, OpenLayers provides a comprehensive set of tools to manipulate maps, overlay data, and interact with geographic information. Its capabilities extend from simple map displays to complex GIS applications, offering you the flexibility to create compelling and interactive mapping solutions. OpenLayers supports a modular and extensible architecture, allowing you to tailor their maps precisely to project requirements.
Explanation of Key Concepts: Maps, Layers, Views, and Sources
Understanding the key concepts within OpenLayers is fundamental to harnessing its full potential:
Map
In OpenLayers, a map is a container for various layers and the view, serving as the canvas where geographical data is displayed. you can create multiple maps within an application, each with its set of layers and views.
The markup below could be used to create a <div>
that contains your map.
<div id="map" style="width: 100%; height: 400px"></div>
The script below constructs a map that is rendered in the <div>
above, using the map id of the element as a selector.
import Map from 'ol/Map.js'; const map = new Map({target: 'map'});
API Doc: ol/Map
View
The view
in OpenLayers determines the center
, zoom
and projection
of the map. It acts as the window through which users observe the geographic data. you can configure different views to represent varying perspectives or zoom levels within a single map.
import View from 'ol/View.js'; map.setView(new View({ center: [0, 0], zoom: 2, }));
The projection determines the coordinate system of the center and the units for map resolution calculations. If not specified (like in the above snippet), the default projection is Spherical Mercator (EPSG:3857), with meters as map units.
The available zoom levels are determined by maxZoom
(default: 28), zoomFactor
(default: 2) and maxResolutio
n (default is calculated in such a way that the projection's validity extent fits in a 256x256 pixel tile).
API Doc: ol/View
Source
Sources provide the data for layers. OpenLayers supports different sources, including Tile sources for raster data, Vector sources for vector data, and Image sources for static images. These sources can fetch data from various providers or be customized to handle specific data formats.
To get remote data for a layer you can use the ol/source
subclasses.
import OSM from 'ol/source/OSM.js'; const source = OSM();
API Doc: ol/source.
Layer
Layers define the visual content of the map. OpenLayers supports various layer types, such as Tile layers for raster data, Vector layers for vector data, and Image layers for rendering images. Layers can be stacked to combine different types of information into a single, coherent map.
ol/layer/Tile
- Renders sources that provide tiled images in grids that are organized by zoom levels for specific resolutions.ol/layer/Image
- Renders sources that provide map images at arbitrary extents and resolutions.ol/layer/Vector
- Renders vector data client-side.ol/layer/VectorTile
- Renders data that is provided as vector tiles.
import TileLayer from 'ol/layer/Tile.js'; // ... const layer = new TileLayer({source: source}); map.addLayer(layer);
API Doc: ol/slayer.
Installing OpenLayers
To start your journey into the world of interactive maps with OpenLayers and React, the first step is to install OpenLayers using your preferred package manager – npm or yarn. Open a terminal and execute one of the following commands:
npm install ol # or yarn add ol
This command fetches the latest version of OpenLayers and installs it as a dependency in your project. With the library now available, you're ready to embark on the next steps of integrating OpenLayers with React.
Setting Up a Basic React Component for the Map
Now that OpenLayers is part of your project, the next crucial step is to create a React component that will serve as the container for your interactive map.
If you try to render the Map before the component has been mounted (meaning outside of useEffect) like following you will get an error message.
const MapComponent = () => { // Incorrect: Rendering content before the component has mounted const map = new Map({ target: 'map', // The ID of the div element where the map will be rendered ... } return <div id="map" style={{ width: '100%', height: '400px' }}></div>; };
Solution:
Ensure that you only render content when the component has properly mounted. You can use lifecycle methods like componentDidMount
in class components or useEffect
in functional components.
const MapComponent = () => { useEffect(() => { // Code here runs after the component has mounted const map = new Map({ target: 'map', // The ID of the div element where the map will be rendered ... } return () => map.setTarget(null) }, []); return <div id="map" style={{ width: '100%', height: '400px' }}></div>; };
The return function will reponsible for resource cleanup for the map.
So a basic OpenLayers React example could look like the following:
// MapComponent.js import React, { useState, useEffect, useRef } from 'react'; import { Map, View } from 'ol'; import TileLayer from 'ol/layer/Tile'; import OSM from 'ol/source/OSM'; import 'ol/ol.css'; function MapComponent() { useEffect(() => { const osmLayer = new TileLayer({ preload: Infinity, source: new OSM(), }) const map = new Map({ target: "map", layers: [osmLayer], view: new View({ center: [0, 0], zoom: 0, }), }); return () => map.setTarget(null) }, []); return ( <div style={{height:'300px',width:'100%'}} id="map" className="map-container" /> ); } export default MapComponent;
In this example, the MapComponent
initializes an OpenLayers map with a simple OpenStreetMap layer and the useEffect
hook ensures that the map is created when the component mounts.
To ensure the correct styling and functionality of OpenLayers, it's crucial to import the necessary CSS and modules. In the MapComponent.js
file, notice the import statement for the OpenLayers CSS:
import 'ol/ol.css'; // Import OpenLayers CSS
This line imports the essential stylesheets required for OpenLayers to render properly. Additionally, other modules from OpenLayers, such as Map, View, TileLayer, and OSM, are imported to create the map instance and layers.
By following these steps, you've successfully set up a basic React component housing an OpenLayers map. You're now ready to delve deeper into the capabilities of OpenLayers and explore advanced features for creating dynamic and interactive maps within your React applications.
Also I created two examples for React and Openlayers:
- OpenLayers 6 React example using a functional component
- OpenLayers 6 React example using a class component
Markers, Popups, and Custom Overlays
Markers, popups, and custom overlays enhance the visual storytelling capabilities of a map, providing users with valuable context. OpenLayers simplifies the process of adding these elements:
- Markers: Representing specific points of interest on a map becomes intuitive with markers. you can add markers to highlight locations, making the map more informative and engaging.
- Popups: Interactive popups can be attached to markers, providing additional information when users click on specific map features. This allows for a more detailed exploration of the data.
- Custom Overlays: OpenLayers allows you to create custom overlays, enabling the display of additional information in a tailored manner. This could include tooltips, legends, or any other supplementary elements.
Here's a simplified example demonstrating the addition of a marker with a popup:
// MarkerPopupMap.js import React, { useEffect } from "react" import Point from 'ol/geom/Point.js'; import "ol/ol.css" import Map from "ol/Map" import View from "ol/View" import {OGCMapTile, Vector as VectorSource} from 'ol/source.js'; import {Tile as TileLayer, Vector as VectorLayer} from 'ol/layer.js'; import Overlay from "ol/Overlay" import {toLonLat} from 'ol/proj.js'; import {toStringHDMS} from 'ol/coordinate.js'; import Feature from 'ol/Feature.js'; const MarkerPopupMap = () => { useEffect(() => { const iconFeature = new Feature({ geometry: new Point([0, 0]), name: 'Null Island', population: 4000, rainfall: 500, }); const vectorSource = new VectorSource({ features: [iconFeature], }); const vectorLayer = new VectorLayer({ source: vectorSource, }); const rasterLayer = new TileLayer({ source: new OGCMapTile({ url: 'https://maps.gnosis.earth/ogcapi/collections/NaturalEarth:raster:HYP_HR_SR_OB_DR/map/tiles/WebMercatorQuad', crossOrigin: '', }), }); const container = document.getElementById('popup'); const content = document.getElementById('popup-content'); const closer = document.getElementById('popup-closer'); const overlay = new Overlay({ element: container, autoPan: { animation: { duration: 250, }, }, }); const map = new Map({ layers: [rasterLayer, vectorLayer], target: "markerpopupmap", view: new View({ center: [0, 0], zoom: 3, }), overlays: [overlay], }); /** * Add a click handler to the map to render the popup. */ map.on('singleclick', function (evt) { const coordinate = evt.coordinate; const hdms = toStringHDMS(toLonLat(coordinate)); content.innerHTML = '<p>You clicked here:</p><code>' + hdms + '</code>'; overlay.setPosition(coordinate); }); return () => map.setTarget(null) }, []) return ( <div> <div id="markerpopupmap" style={{ width: "100%", height: "400px" }} /> <div id="popup" class="ol-popup" style={{backgroundColor:'#fff'}}> <a href="#" id="popup-closer" class="ol-popup-closer"></a> <div id="popup-content"></div> </div> </div> ) } export default MarkerPopupMap
Handling Map Events and User Interactions:
Interactive maps come to life when you handle events and user interactions effectively. OpenLayers simplifies this process by providing robust event handling mechanisms. Consider the following example demonstrating how to capture a click event on the map:
// Handle a click event on the map map.on('click', (event) => { const clickedCoordinate = event.coordinate; console.log('Clicked Coordinate:', clickedCoordinate); });
This example displays OpenLayers' event handling to log the coordinates of a click event on the map. you can extend this functionality to respond to various user interactions, such as dragging, zooming, or even custom gestures.
useState for Managing State: Use the useState hook to manage state within the React component. This is particularly useful for dynamic changes to the map, such as updating the center or zoom level based on user interactions.
const [mapCenter, setMapCenter] = useState([0, 0]); // Update the map's center based on user interaction const handleMapInteraction = (event) => { const newCenter = event.map.getView().getCenter(); setMapCenter(newCenter); };
Advanced OpenLayers Map Features
Adding Vector Layers and Working with GeoJSON Data
Vector layers in OpenLayers allow you to display and interact with vector data, opening up possibilities for intricate and detailed map representations. Leveraging GeoJSON, a popular format for encoding geographic data, is a common practice. Below is an example of incorporating a vector layer with GeoJSON data into a React component:
// VectorLayerMap.js import React, { useEffect } from 'react'; import 'ol/ol.css'; import Map from 'ol/Map'; import View from 'ol/View'; import TileLayer from 'ol/layer/Tile'; import OSM from 'ol/source/OSM'; import VectorLayer from 'ol/layer/Vector'; import VectorSource from 'ol/source/Vector'; import GeoJSON from 'ol/format/GeoJSON'; const VectorLayerMap = () => { useEffect(() => { const vectorSource = new VectorSource({ features: new GeoJSON().readFeatures(geojsonObject), }); const vectorLayer = new VectorLayer({ source: vectorSource, }); const map = new Map({ target: 'vectorLayerMap', layers: [ new TileLayer({ source: new OSM(), }), vectorLayer, ], view: new View({ center: [0, 0], zoom: 2, }), }); return () => map.setTarget(null) }, []); return <div id="vectorLayerMap" style={{ position: 'relative', width: '100%', height: '400px' }}></div>; }; export default VectorLayerMap; const geojsonObject = { 'type': 'Feature', 'geometry': { 'type': 'MultiLineString', 'coordinates': [ [ [-1e6, -7.5e5], [-1e6, 7.5e5], ], [ [1e6, -7.5e5], [1e6, 7.5e5], ], [ [-7.5e5, -1e6], [7.5e5, -1e6], ], [ [-7.5e5, 1e6], [7.5e5, 1e6], ], ], } }
Optimizing React and OpenLayers Integration: Strategies for Rendering Performance
1. Addressing Rendering Performance Concerns:
Efficient rendering is paramount in any web application, and integrating OpenLayers with React requires careful consideration of performance concerns. Here are some strategies to address rendering performance:
-
Debouncing and Throttling: When handling events that trigger frequent updates, such as map movements or zoom changes, implement debouncing or throttling techniques. This prevents excessive re-renders and ensures that updates are processed at a controlled rate.
-
Batched State Updates: Use React's
setState
batching mechanism to group multiple state updates into a single render cycle. This reduces the number of renders triggered by multiple state changes, resulting in a more efficient rendering process.
2. Implementing Lazy Loading for Map Components:
To enhance overall application performance, especially in scenarios where maps are not initially visible or are part of larger applications, consider implementing lazy loading for map components. This ensures that the OpenLayers library and associated map components are only loaded when needed.
- Dynamic Imports: Use dynamic imports and React's
React.lazy
to load OpenLayers and map components lazily. This approach allows you to split your code into smaller chunks that are loaded on-demand, reducing the initial page load time.
// Example using React.lazy const LazyLoadedMap = React.lazy(() => import('./LazyLoadedMap')); const App = () => ( <div> {/* Other components */} <React.Suspense fallback={<div>Loading...</div>}> <LazyLoadedMap /> </React.Suspense> </div> );
3. Memoization Techniques Using React Hooks:
Memoization is a powerful technique to optimize expensive calculations and prevent unnecessary renders. React provides hooks like useMemo
and useCallback
for effective memoization.
useMemo: Use useMemo
to memoize the result of a computation and ensure that it is only recalculated when dependencies change. This is particularly useful when dealing with derived data or complex computations within your map components.
const expensiveData = /* some expensive computation */; const MyMapComponent = ({ center, zoom }) => { const memoizedData = React.useMemo(() => expensiveData, [center, zoom]); // Component logic using memoizedData... };
useCallback: When passing functions as props to child components, use useCallback
to memoize those functions. This ensures that the same function reference is maintained across renders unless its dependencies change.
const MyMapComponent = ({ onMapClick }) => { const handleClick = React.useCallback(() => { // Handle map click... onMapClick(); }, [onMapClick]); // Component logic using handleClick... };
These practices contribute to a more responsive and optimized integration of OpenLayers within React applications, enhancing the overall user experience.
For additional inspiration and examples, explore the OpenLayers API Documentation. You can also find valuable examples specific to React and OpenLayers at https://codesandbox.io/examples/package/react-openlayers.
Resources:
Table of contents
- Overview of OpenLayers and its Capabilities
- Explanation of Key Concepts: Maps, Layers, Views, and Sources
- Installing OpenLayers
- Setting Up a Basic React Component for the Map
- Markers, Popups, and Custom Overlays
- Handling Map Events and User Interactions:
- Advanced OpenLayers Map Features
- Optimizing React and OpenLayers Integration: Strategies for Rendering Performance
First published February 22, 2024
Have you published a response to this? Send me a webmention by letting me know the URL.
Found no Webmentions yet. Be the first!
About The Author

Geospatial Developer
Hi, I'm Max (he/him). I am a geospatial developer, author and cyclist from Rosenheim, Germany. Support me