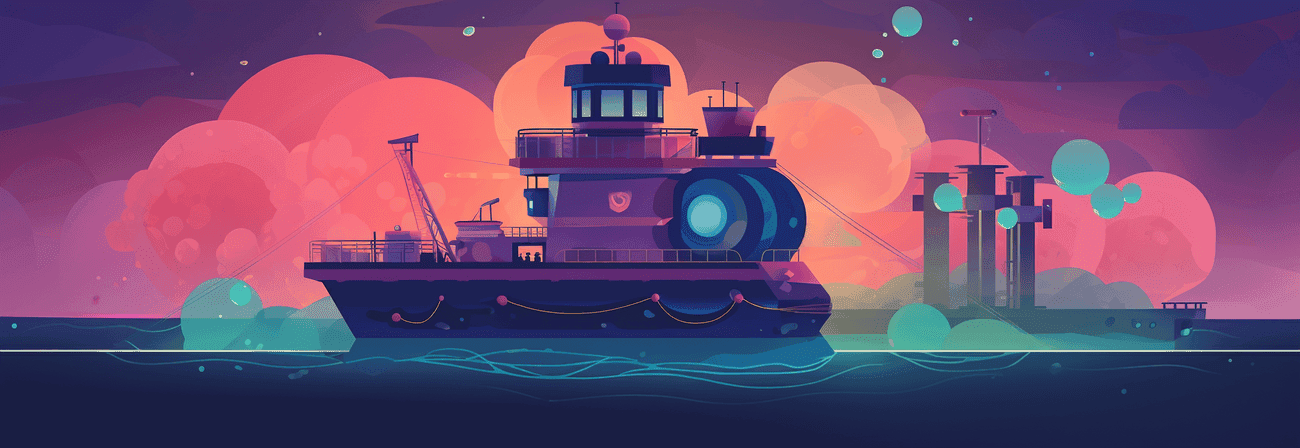
Dockerizing a Next.js Application with GitHub Actions
In this article, we'll explore how to Dockerize a Next.js application and automate its deployment using GitHub Actions, thereby simplifying the deployment workflow and enhancing development productivity.
Prerequisites
Before we dive into Dockerizing our Next.js application and setting up GitHub Actions for deployment, ensure you have the following prerequisites:
- A Next.js project.
- Docker installed on your local machine.
- A GitHub repository for your Next.js project.
Setting up Docker
Docker allows you to package your application and its dependencies into a container, ensuring consistency across different environments. Start by creating a Dockerfile
in the root of your Next.js project:
FROM node:18-alpine AS base # Install dependencies only when needed FROM base AS deps # Check https://github.com/nodejs/docker-node/tree/b4117f9333da4138b03a546ec926ef50a31506c3#nodealpine to understand why libc6-compat might be needed. RUN apk add --no-cache libc6-compat WORKDIR /app # Install dependencies based on the preferred package manager COPY package.json yarn.lock* package-lock.json* pnpm-lock.yaml* ./ RUN \ if [ -f yarn.lock ]; then yarn --frozen-lockfile; \ elif [ -f package-lock.json ]; then npm ci; \ elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm i --frozen-lockfile; \ else echo "Lockfile not found." && exit 1; \ fi # Rebuild the source code only when needed FROM base AS builder WORKDIR /app COPY --from=deps /app/node_modules ./node_modules COPY . . # Next.js collects completely anonymous telemetry data about general usage. # Learn more here: https://nextjs.org/telemetry # Uncomment the following line in case you want to disable telemetry during the build. # ENV NEXT_TELEMETRY_DISABLED 1 RUN \ if [ -f yarn.lock ]; then yarn run build; \ elif [ -f package-lock.json ]; then npm run build; \ elif [ -f pnpm-lock.yaml ]; then corepack enable pnpm && pnpm run build; \ else echo "Lockfile not found." && exit 1; \ fi # Production image, copy all the files and run next FROM base AS runner WORKDIR /app ENV NODE_ENV production # Uncomment the following line in case you want to disable telemetry during runtime. # ENV NEXT_TELEMETRY_DISABLED 1 RUN addgroup --system --gid 1001 nodejs RUN adduser --system --uid 1001 nextjs COPY --from=builder /app/public ./public # Set the correct permission for prerender cache RUN mkdir .next RUN chown nextjs:nodejs .next # Automatically leverage output traces to reduce image size # https://nextjs.org/docs/advanced-features/output-file-tracing COPY --from=builder --chown=nextjs:nodejs /app/.next/standalone ./ COPY --from=builder --chown=nextjs:nodejs /app/.next/static ./.next/static USER nextjs EXPOSE 3000 ENV PORT 3000 # set hostname to localhost ENV HOSTNAME "0.0.0.0" # server.js is created by next build from the standalone output # https://nextjs.org/docs/pages/api-reference/next-config-js/output CMD ["node", "server.js"]
This Dockerfile is the default Dockerfile provided by Vercel to set up a Node.js environment, install dependencies, build the Next.js application, and exposing port 3000.
You have to ensure you are using output: "standalone"
in your next.config.js
.
const nextConfig = { output: "standalone", }
Before proceeding further, it's crucial to test our Dockerized Next.js application locally to ensure everything functions as expected. Open a terminal in the project directory and execute the following commands:
# Build the Docker image docker build -t my-nextjs-app . # Run the Docker container docker run -p 3000:3000 my-nextjs-app
Visit http://localhost:3000
in your web browser to verify that your Next.js application is running within the Docker container.
Setting up GitHub Actions for Continuous Deployment:
GitHub Actions automate the CI/CD pipeline directly from your GitHub repository. Create a .github/workflows/pipeline.yml
file with the following content:
name: Docker Build & Publish on: push: branches: [main] jobs: build: name: Build Docker runs-on: ubuntu-latest steps: - name: Check out repository code 🛎️ uses: actions/checkout@v4 - name: Set up Docker Buildx 🚀 uses: docker/setup-buildx-action@v3 - name: Login to Docker Hub 🚢 uses: docker/login-action@v3 with: username: ${{ secrets.DOCKER_HUB_USERNAME}} password: ${{ secrets.DOCKER_HUB_ACCESS_TOKEN}} - name: Build and push 🏗️ uses: docker/build-push-action@v2 with: context: . file: ./Dockerfile push: true tags: | ${{ secrets.DOCKER_HUB_USERNAME}}/{docker_repository}:${{ github.sha }} ${{ secrets.DOCKER_HUB_USERNAME}}/{docker_repository}:latest
Replace ${{ secrets.DOCKER_USERNAME }}
and ${{ secrets.DOCKER_PASSWORD }}
with your Docker Hub username and password/access token and {docker_repository}
with the name of your Docker repository. These secrets should be stored securely in your repository's settings.
This workflow will build your container from your GitHub repositiory and push it to your Docker COntainer registry with two tags:
:latest
and:{commitID}
Passing environment variables to the Workflow
In case you need some environment variables you have to adjust the Dockerfile
with some additional parameters. To be able to use environment variables which are stored in your repository as secrets you will need to mount and export every environment variable like the following to your npm run build
command.
RUN --mount=type=secret,id=NEXT_PUBLIC_CMS_URL \ export NEXT_PUBLIC_CMS_URL=$(cat /run/secrets/NEXT_PUBLIC_CMS_URL) && \ npm run build
You can have a look at the Dockerfile for my site for a example: personal website Dockerfile.
Also you will need to modify the step Build and push
in the workflow like this:
- name: Build and push 🏗️ uses: docker/build-push-action@v2 with: context: . file: ./Dockerfile push: true tags: | ${{ secrets.DOCKER_HUB_USERNAME}}/personal-website:${{ github.sha }} ${{ secrets.DOCKER_HUB_USERNAME}}/personal-website:latest secrets: | "NEXT_PUBLIC_STRAPI_API_URL=${{ secrets.NEXT_PUBLIC_CMS_URL }}"
Conclusion
With this setup, every push to the main branch of your GitHub repository triggers the CI/CD pipeline. Continuous Integration and Continuous Deployment for Dockerized Next.js applications provide a streamlined and efficient development process, ensuring that your application is always in a deployable state. By combining GitHub Actions with Docker, you can automate the deployment process and focus on building and improving your Next.js application.
First published February 6, 2024
Have you published a response to this? Send me a webmention by letting me know the URL.
Found no Webmentions yet. Be the first!
About The Author

Geospatial Developer
Hi, I'm Max (he/him). I am a geospatial developer, author and cyclist from Rosenheim, Germany. Support me